 |
 | |
ASP DataGridColumns .NET assembly (for VB.NET, C#, J#)
|
Obviously, your task is to create a database connection and command that binds data to an ASP .NET DataGrid server control. Also you need provide your users with a friendly interface.
One of the most permanent challenges in designing user interfaces is figuring out how to display large amounts of data efficiently and intuitively without bewildering the user. The problem becomes particularly thorny when the interface must give the ability to easily update and store the data that the user needs to modify.
The ASP .NET DataGrid control gives developers a powerful and flexible tool to meet this challenge. You do not need to look for some third-vendors’ costly datagrids. The ASP .NET DataGrid server control is smart enough.
|
|
ASPDataGridColumns .NET assembly from RustemSoft is a DataGrid Columns software package specifically designed for ASP .NET developers. The assembly allows you to use all strengths of the .NET DataGrid server control without waiving the user interface elements your customers need.
|
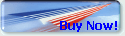 |
|
The downloadable samples explain ASP .NET DataGrid control basic operations and show how to extend it to display different kinds of data columns in an application-appropriate manner.
By using the assembly you can create your own set of DataGrid Columns objects that defines custom columns for the ASP .NET DataGrid server control.
A column is an object that defines what the column looks and behaves like, including such things as color, font, and the presence of controls that will handle linked field in a database with the use of a Combo Box, a Check Box and other control.
The RustemSoft ASP DataGrid Columns assembly contains a special  DataGridCommands class that allows you to manage a DataGrid's datasource. The ASPDataGridColumns dynamic link library contains the following DataGrid Columns:
|
|
VB .NET
' Save datagrid data source tableDataSource table
Dim DGCommands As DataGridCommands = New DataGridCommands()
DGCommands.UpdateDataSource(tableDataSource, yourDataGrid)
C#
// Save datagrid data source tableDataSource table
DataGridCommands DGCommands = new DataGridCommands();
DGCommands.UpdateDataSource(tableDataSource, yourDataGrid);
|
|
|
By clicking the "Save" button your user will be able to save all updates into DataGrid's datasource data object.
|
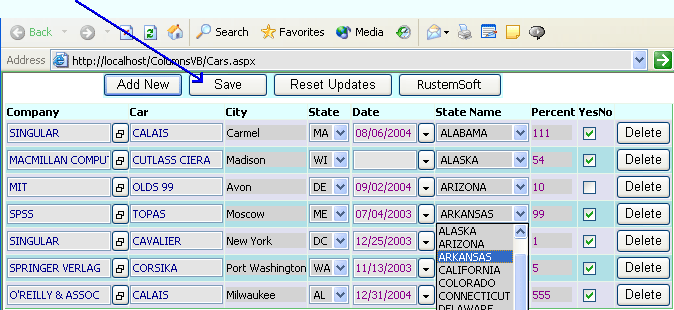 |
|
VB .NET
' Define comboCompany variable as an object of ComboBoxColumn class
' and assign it to the DataGrid1 'Company' column.
Dim comboCompany As ComboBoxColumn = DataGrid1.Columns(5)
' Define Name of Interface Page that will be called by clicking on ComboBox button
comboCompany.InterfacePageName = "ComboInterfacePage.aspx"
|
' Define width of Interface Page
comboCompany.InterfacePageWidth = 290
' Define height of Interface Page
comboCompany.InterfacePageHeight = 400
' Assign DataSource property for comboCompany object as tblCompanies
' data table where Companys’s names are stored.
comboCompany.DataSource = tblCompanies
' Specify DisplayMember as "Name" field of tblCompanies table
comboCompany.DisplayMember = "Name"
' Specify ValueMember as "PubID" field of tblCompanies table
comboCompany.ValueMember = "PubID"
' Identify "Company" column's foreground color
comboCompany.ForeColor = Color.DarkMagenta
' Identify "Company" column characters' font and size
comboCompany.Font_Name = "Tahoma"
comboCompany.Font_Size = FontUnit.Point(8)
' Adjust "Company" column's width
comboCompany.Width = Unit.Point(60)
C#
// Define comboCompany variable as an object of ComboBoxColumn class
// and assign it to the DataGrid1 'Company' column.
ComboBoxColumn comboCompany = (ComboBoxColumn)DataGrid1.Columns[5];
|
|
|
// Define Name of Interface Page that will be called by clicking on ComboBox button
comboCompany.InterfacePageName = "ComboInterfacePage.aspx";
// Define width of Interface Page
comboCompany.InterfacePageWidth = 345;
// Define height of Interface Page
comboCompany.InterfacePageHeight = 400;
// Assign DataSource property for comboCompany object as tblCompanies
// data table where Companys’s names are stored.
comboCompany.DataSource = tblCompanies;
// Specify DisplayMember as "Name" field of tblCompanies table
comboCompany.DisplayMember = "Name";
// Specify ValueMember as "PubID" field of tblCompanies table
comboCompany.ValueMember = "PubID";
// Identify "Company" column's foreground color
comboCompany.ForeColor = Color.DarkMagenta;
// Identify "Company" column characters' font and size
comboCompany.Font_Name = "Tahoma";
comboCompany.Font_Size = FontUnit.Point(8);
// Adjust "Company" column's width
comboCompany.Width = Unit.Point(60);
|
 Syntax
<RustemSoft:TextBoxColumn DataField="Data Field Name" HeaderText="Header Text"/>
|
VB .NET
' Define TxtBox variable as an object of TextBox Column class
' and assign it to the DataGrid1 "City" column.
Dim TxtBox As TextBoxColumn = DataGrid1.Columns(2)
' Identify "City" column's foreground color
TxtBox.ForeColor = Color.DarkMagenta
' Identify "City" column characters' font and size
TxtBox.Font_Name = "Tahoma"
TxtBox.Font_Size = FontUnit.Point(8)
' Adjust "City" column's width
TxtBox.Width = Unit.Point(60)
C#
// Define TxtBox variable as an object of TextBox Column class
// and assign it to the DataGrid1 "City" column.
TextBoxColumn TxtBox = (TextBoxColumn)DataGrid1.Columns[2];
// Identify "City" column's foreground color
TxtBox.ForeColor = Color.DarkMagenta;
// Identify "City" column characters' font and size
TxtBox.Font_Name = "Tahoma";
TxtBox.Font_Size = FontUnit.Point(8);
// Adjust "City" column's width
TxtBox.Width = Unit.Point(60);
|
|
|
VB .NET
' Define DropDown variable as an object of DropDownColumn class
' and assign it to the DataGrid1 'State' column.
Dim DropDown As DropDownColumn = DataGrid1.Columns(5)
' Assign DataSource property for DropDown object as tblStates
' data table where states’s names are stored.
DropDown.DataSource = tblStates
' Specify DisplayMember as "Name" field of tblStates table
DropDown.DisplayMember = "Name"
' Specify ValueMember as "State" field of tblStates table
DropDown.ValueMember = "State"
' Identify "State" column's foreground color
DropDown.ForeColor = Color.DarkMagenta
' Identify "State" column characters' font and size
DropDown.Font_Name = "Tahoma"
DropDown.Font_Size = FontUnit.Point(8)
' Adjust 'State' column's width
DropDown.Width = Unit.Point(60)
C#
// Define DropDown variable as an object of DropDownColumn class
// and assign it to the DataGrid1 'State' column.
DropDownColumn DropDown = (DropDownColumn)DataGrid1.Columns[5];
// Assign DataSource property for DropDown object as tblStates
// data table where states’s names are stored.
DropDown.DataSource = tblStates;
// Specify DisplayMember as "Name" field of tblStates table
DropDown.DisplayMember = "Name";
// Specify ValueMember as "State" field of tblStates table
DropDown.ValueMember = "State";
// Identify "State" column's foreground color
DropDown.ForeColor = Color.DarkMagenta;
// Identify "State" column characters' font and size
DropDown.Font_Name = "Tahoma";
DropDown.Font_Size = FontUnit.Point(8);
// Adjust 'State' column's width
DropDown.Width = Unit.Point(60);
|
|
|
VB .NET
' Define DatePicker variable as an object of DatePickerColumn class
' and assign it to the DataGrid1 'Date' column.
Dim DatePicker As DatePickerColumn = DataGrid1.Columns(5)
' Identify "Date" column's foreground color
DatePicker.ForeColor = Color.DarkMagenta
' Identify "Date" column characters' font and size
DatePicker.Font_Name = "Tahoma"
DatePicker.Font_Size = FontUnit.Point(8)
' Adjust 'Date' column's width
DatePicker.Width = Unit.Point(60)
' Define yearGreaterThanPresentYear property. If the inserted year is greater
' than current year plus 20 the control will use "19" as first two digits for year,
' otherwise will use "20"
DatePicker.yearGreaterThanPresentYear = 20
C#
// Define DatePicker variable as an object of DatePickerColumn class
// and assign it to the DataGrid1 'Date' column.
DatePickerColumn DatePicker = (DatePickerColumn)DataGrid1.Columns[5];
// Identify "Date" column's foreground color
DatePicker.ForeColor = Color.DarkMagenta;
// Identify "Date" column characters' font and size
DatePicker.Font_Name = "Tahoma";
DatePicker.Font_Size = FontUnit.Point(8);
// Adjust 'Date' column's width
DatePicker.Width = Unit.Point(60);
// Define yearGreaterThanPresentYear property. If the inserted year is greater
// than current year plus 20 the control will use "19" as first two digits for year,
// otherwise will use "20"
DatePicker.yearGreaterThanPresentYear = 20
|
|
 ASP DataGrid CheckBox Column
VB .NET
' Define CheckBox variable as an object of CheckBox Column class
' and assign it to the DataGrid1 'YesNo' column.
Dim CheckBox As CheckBox Column = DataGrid1.Columns(5)
C#
// Define CheckBox variable as an object of CheckBox Column class
// and assign it to the DataGrid1 'YesNo' column.
CheckBox Column CheckBox = (CheckBox Column)DataGrid1.Columns[5];
|
|
 ASP DataGrid DateTime Column
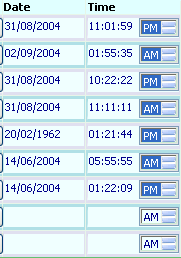 | |
You have a DataGrid with a DateTime field that displays the date/time as "2/20/2005 7:00:00 PM". You would like to display only the time in the following format "7:00 PM". You would like to insert a new time in the column or update current time value only. Let us suppose that you are European and would like to see in date field something like "20.02.2005". The ASP DataGrid DateTime Column can help you design the concept that will resolve your needs.
The DateTime Column is used to allow the user to insert formatted date and time, and to display that date/time in your ASP DataGrid server control interface.
|
 The ASP DataGrid DateTime Column class has the following useful properties:
Format - Various formats may be set. You may use a date/time format (like MMDDYY, MMDDYYYY, DDMMYY, DDMMYYYY, YYYYMMDD, HHMM12, HHMMSS12, HHMM24, HHMMSS24) to enable the date/time inserting and updating. Default is MMDDYYYY.
DefaultValue - any DateTime value. For example, you can set the DefaultValue to today's date. Default is empty DateTime (null/Nothing).
DelimiterChar - a separator between date/time fractions (between days and month or hours and minutes). Default is "/".
|
|
VB .NET
' Define DateTime variable as an object of DateTimeColumn class
' and assign it to the DataGrid1 'Time' column.
Dim DateTime As DateTimeColumn = DataGrid1.Columns(5)
' Define Time Format
DateTime.Format = DateTime.DateTimeFormats.HHMMSS12
' Define separator char
DateTime.DelimiterChar = ":"
C#
// Define DateTime variable as an object of DateTimeColumn class
// and assign it to the DataGrid1 'Time' column.
DateTime ColumnDateTime = (DateTimeColumn)DataGrid1.Columns[5];
// Define Time Format
DateTime.Format = DateTime.DateTimeFormats.HHMMSS12;
// Define separator char
DateTime.DelimiterChar = ":";
|
|
|
 How to turn off error messages? How to define error messages in my native language?
VB .NET
' You may turn error messages off
DateTime.ErrMessageVisible = False
' You can specify an error message in your native language:
DateTime.ErrMessage = "Date Inadmissible ! Réintroduisez svp."
C#
// You may turn error messages off
DateTime.ErrMessageVisible = false;
// You can specify an error message in your native language:
DateTime.ErrMessage = "Date Inadmissible ! Réintroduisez svp.";
|
|
|
 ASP DataGrid Numeric Column
VB .NET
' Define Percent variable as an object of NumericColumn class
' and assign it to the DataGrid1 'Percent' column.
Dim Percent As NumericColumn = DataGrid1.Columns(5)
' Identify 'Percent' column's foreground color
Percent.ForeColor = Color.DarkMagenta
' Identify 'Percent' column characters' font and size
Percent.Font_Name = "Tahoma"
Percent.Font_Size = FontUnit.Point(8)
' Adjust 'Percent' column's width
Percent.Width = Unit.Point(60)
' Define that Percent column accepts positive values only
Percent.PositiveOnly = True
' Define Percent column’s max length
Percent.MaxLength = 8
' Define Percent column’s Decimal Length
Percent.DecimalLength = 2
' Define Percent column’s max possible value
Percent.MaxValue = 10000
' Define Percent column’s min possible value
Percent.MinValue = 100
' Define Percent column’s error message for max value
Percent.ErrMessageMaxValue = "Too much!"
C#
// Define Percent variable as an object of NumericColumn class
// and assign it to the DataGrid1 'Percent' column.
NumericColumn Percent = (NumericColumn)DataGrid1.Columns[5];
// Identify 'Percent' column's foreground color
Percent.ForeColor = Color.DarkMagenta;
// Identify 'Percent' column characters' font and size
Percent.Font_Name = "Tahoma";
Percent.Font_Size = FontUnit.Point(8);
// Adjust 'Percent' column's width
Percent.Width = Unit.Point(60);
// Define that Percent column accepts positive values only
Percent.PositiveOnly = true;
// Define Percent column’s max length
Percent.MaxLength = 8;
// Define Percent column’s Decimal Length
Percent.DecimalLength = 2;
// Define Percent column’s max possible value
Percent.MaxValue = 10000;
// Define Percent column’s min possible value
Percent.MinValue = 100;
// Define Percent column’s error message for max value
Percent.ErrMessageMaxValue = "Too much!";
|
|
|
 How to turn off error messages? How to define error messages in my native language?
VB .NET
' You may turn error messages off
Percent.ErrMessageVisible = False
' You can specify an error message in your native language:
Percent.ErrMessage = "Date Inadmissible ! Réintroduisez svp."
C#
// You may turn error messages off
Percent.ErrMessageVisible = false;
// You can specify an error message in your native language:
Percent.ErrMessage = "Date Inadmissible ! Réintroduisez svp.";
|
|
 ASP DataGrid Text Fractions Column
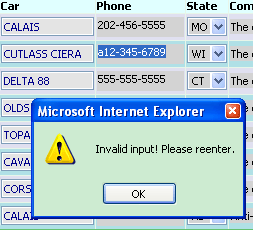 | |
This formatted intelligent ASP .NET DataGrid Text Fractions Column can mask the text fractions. It gives you ability to manage the IP Address, SS#, Phone numbers, etc., and checks the validation, and automatically set the delimiter location.
The TextFractionsColumn class is useful in several situations. For example, this control will automatically format a telephone number so when a user enters their phone number like this:
123-456-7890
or
1234567890
it will automatically change the numbers in the column text updating box.
Up to 5 text fractions can be defined. By setting the DelimiterChar property up a delimiter symbol for the control can be specified. You can define the overall length of each text fraction and what kind of characters can be inserted in the fraction (alphanumeric, numeric only, etc.).
|
|
VB .NET
' Define PhoneColumn variable as an object of TextFractionsColumn class
' and assign it to the DataGrid1 'Phone' column.
Dim PhoneColumn As TextFractionsColumn = DataGrid1.Columns(5)
' Identify 'Phone' column's foreground color
PhoneColumn.ForeColor = Color.DarkMagenta
' Identify 'Phone' column characters' font and size
PhoneColumn.Font_Name = "Tahoma"
PhoneColumn.Font_Size = FontUnit.Point(8)
' Adjust 'Phone' column's width
PhoneColumn.Width = Unit.Point(60)
' Specify Delimiter Character for the field as space
PhoneColumn.DelimiterChar = " "
' Specify first fraction properties
' Alphanumeric symbols only are acceptable for the fraction
PhoneColumn.FractionsCode(0, 0) = "a"
' You can insert 3 symbols only into the first fraction
PhoneColumn.FractionsCode(0, 1) = 3
' Specify second fraction properties
' Numeric symbols only are acceptable for the fraction
PhoneColumn.FractionsCode(1, 0) = "n"
' You can insert 3 symbols only into the second fraction
PhoneColumn.FractionsCode(1, 1) = 3
' Specify third fraction properties
' Numeric symbols only are acceptable for the fraction
PhoneColumn.FractionsCode(2, 0) = "n"
' You can insert 4 symbols only into the third fraction
PhoneColumn.FractionsCode(2, 1) = 4
' Define PhoneColumn column’s error message for max value
PhoneColumn.ErrMessageMaxValue = "Not correct phone #!"
C#
// Define PhoneColumn variable as an object of TextFractionsColumn class
// and assign it to the DataGrid1 'Phone' column.
TextFractionsColumn PhoneColumn = (TextFractionsColumn)DataGrid1.Columns[5];
// Identify 'Phone' column's foreground color
PhoneColumn.ForeColor = Color.DarkMagenta;
// Identify 'Phone' column characters' font and size
PhoneColumn.Font_Name = "Tahoma";
PhoneColumn.Font_Size = FontUnit.Point(8);
// Adjust 'Phone' column's width
PhoneColumn.Width = Unit.Point(60);
// Specify Delimiter Character for the field as space
PhoneColumn.DelimiterChar = " ";
// Specify first fraction properties
// Alphanumeric symbols only are acceptable for the fraction
PhoneColumn.FractionsCode[0, 0) = "a";
// You can insert 3 symbols only into the first fraction
PhoneColumn.FractionsCode[0, 1] = 3;
// Specify second fraction properties
// Numeric symbols only are acceptable for the fraction
PhoneColumn.FractionsCode[1, 0] = "n";
// You can insert 3 symbols only into the second fraction
PhoneColumn.FractionsCode[1, 1] = 3;
// Specify third fraction properties
// Numeric symbols only are acceptable for the fraction
PhoneColumn.FractionsCode[2, 0] = "n";
// You can insert 4 symbols only into the third fraction
PhoneColumn.FractionsCode[2, 1] = 4;
// Define PhoneColumn column’s error message for max value
PhoneColumn.ErrMessageMaxValue = "Not correct phone #!";
|
|
|
 How to turn off error messages? How to define error messages in my native language?
VB .NET
' You may turn error messages off
PhoneColumn.ErrMessageVisible = False
' You can specify an error message in your native language:
PhoneColumn.ErrMessage = "Date Inadmissible ! Réintroduisez svp."
C#
// You may turn error messages off
PhoneColumn.ErrMessageVisible = false;
// You can specify an error message in your native language:
PhoneColumn.ErrMessage = "Date Inadmissible ! Réintroduisez svp.";
|
 ASP DataGrid Text Edit Column
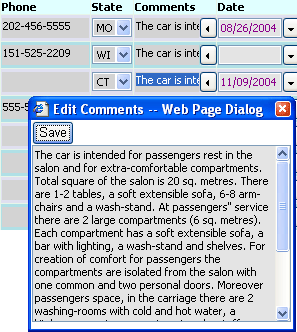 | |
The RustemSoft ASP DataGrid TextEdit Column gives you a useful TextEdit Field control, which presents an edit window when user selects a cell of the ASP DataGrid TextEdit Column on .NET DataGrid. This provides a pop-up TextEdit field editor that you can call from your code at any time to let users edit the text in a TextEdit field.
This Text Edit field editor provides more flexibility by updating the TextEdit field to be used in an ASP .NET DataGrid server control's column.
The ASP DataGrid Text Edit Column TextEdit field editor is useful for showing short document contents, like Memos and emails.
When using the DataGrid TextEdit Column editor you are automatically provided with all general features, which any typical, contemporary word processor has.
|
|
 ASP DataGrid UpDown Column
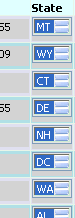 |
|
The RustemSoft ASP DataGrid UpDown Column represents a datagrid column up-down control that displays a list's selected values. It allows you to show a UpDown control in a Datagrid cell. The control displays in a datagrid cell a single value that is selected from a collection by clicking the up or down buttons of the control. The user can also enter text in the control if the string typed in matched an item in the collection to be accepted.
To create a collection of objects to display in the UpDown control, you can add or remove the items individually by using the Add and Remove methods.
You can identify a DataSource parameter for the control. The DataSource is a source for DataGrid UpDown column values list as System.Data.DataTable or System.Data.DataRowView or System.Collections.ArrayList. By defining DisplayMember parameter you can specify a field to display in UpDown column as String (name of table column).
Use the ASP DataGrid UpDownColumn to create a single selection up-down list control in each cell of ASP .NET DataGrid column. You can control the appearance of the ASP DataGrid UpDownColumn by setting the BackColor, ForeColor, BorderColor, BorderStyle, and other properties.
The ASP DataGrid UpDownColumn supports data binding. To bind the control to a data source, create a data source, such as a System.Collections.ArrayList object, that contains the items to display in the column. Then, use the DataSource property to bind the data source to the ASP DataGrid UpDownColumn. You can use a DataTable object as a datasource for your UpDownColumn. For this kind of a datasource you need to specify ValueMember and DisplayMember properties.
|
VB .NET
' Define StateColumn variable as an object of UpDownColumn class
' and assign it to the DataGrid1 'State' column.
Dim StateColumn As UpDownColumn = DataGrid1.Columns(3)
Dim al As ArrayList = New ArrayList
SQL = "SELECT * FROM States"
DA = New System.Data.OleDb.OleDbDataAdapter(SQL, conStr)
Dim tblStates As DataTable = New DataTable
DA.Fill(tblStates)
Dim row As DataRow
' Populate tblStates table's records into al ArrayList
For Each row In tblStates.Rows
al.Add(row("State"))
Next
StateColumn.DataSource = al
' Identify “State” column’s background color
StateColumn.BackColor = Color.LightGray
' Identify “State” column characters’ font and size
StateColumn.Font_Name = "Tahoma"
StateColumn.Font_Size = FontUnit.Point(8)
' Adjust ‘State’ column’s width
StateColumn.Width = Unit.Point(30)
C#
// Define StateColumn variable as an object of UpDownColumn class
// and assign it to the DataGrid1 'State' column.
UpDownColumn StateColumn = (UpDownColumn)DataGrid1.Columns[3];
ArrayList al = new ArrayList();
SQL = "SELECT * FROM States";
DA = new System.Data.OleDb.OleDbDataAdapter(SQL, conStr);
DataTable tblStates = new DataTable();
DA.Fill(tblStates);
// Populate tblStates table's records into al ArrayList
foreach (DataRow row in tblStates.Rows)
{
al.Add(row["State"]);
}
StateColumn.DataSource = al;
// Identify “State” column’s background color
StateColumn.BackColor = Color.LightGray;
// Identify “State” column characters’ font and size
StateColumn.Font_Name = "Tahoma";
StateColumn.Font_Size = FontUnit.Point(8);
// Adjust ‘State’ column’s width
StateColumn.Width = Unit.Point(30);
|
|
|
|